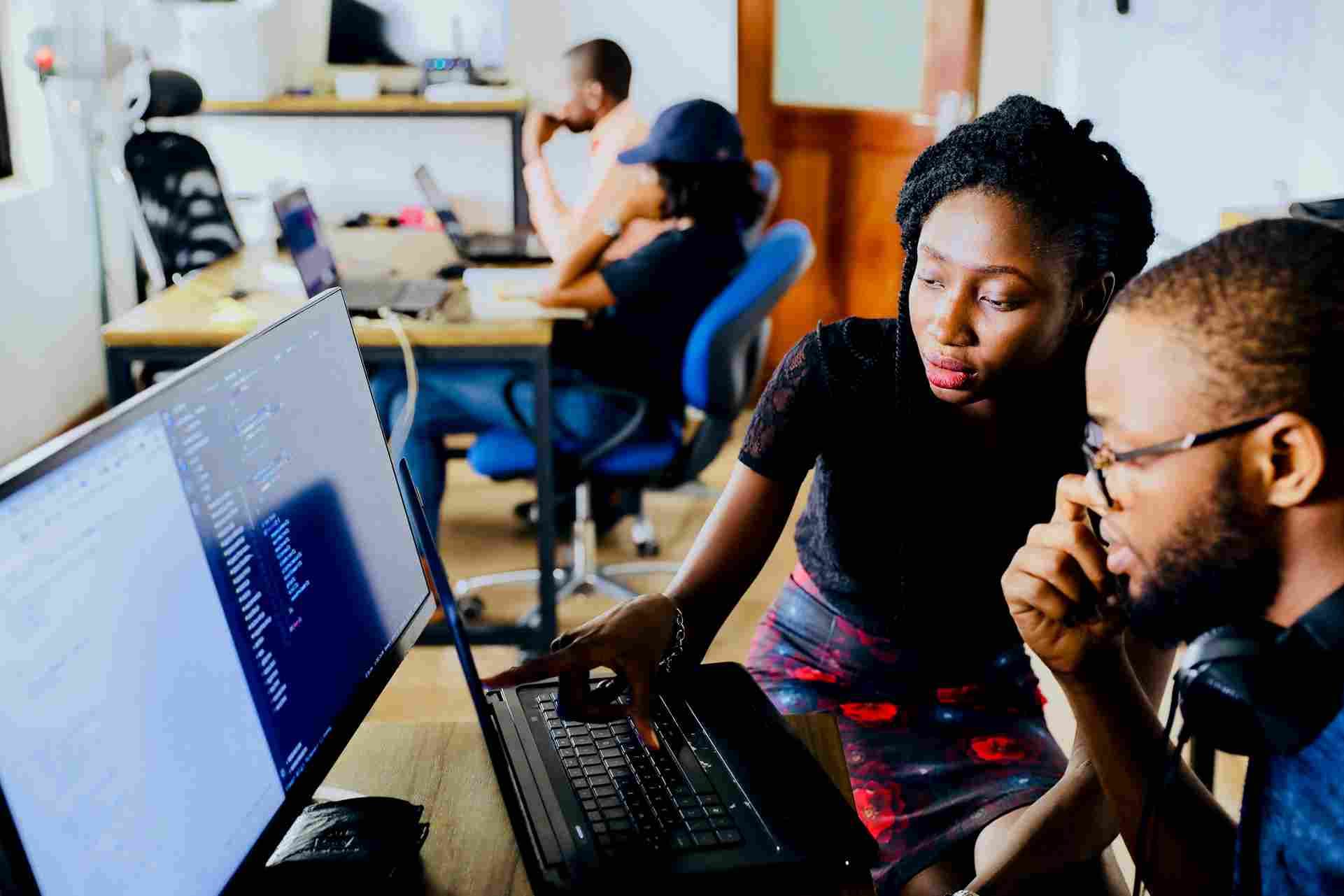
How to Manage State in Next.js for Optimal Performance
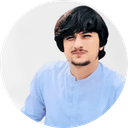
malik
08/01/2025
State control is one of the most crucial aspects of building scalable and performant net packages, and Next.Js 15 offers loads of equipment and techniques to deal with it successfully. Whether you're managing worldwide kingdom, local issue kingdom, or server-facet state, know-how the proper approach can drastically enhance your application's performance and maintainability.
In this guide, we'll explore the core principles of how to manage state in Next.js, covering the latest techniques, tools, and best practices. From client-side to server-side state management, you'll learn how to strike the right balance for a seamless user experience.
you may have a deep expertise of nation control techniques in Next.Js 15 and practical suggestions to optimize your application's overall performance.
Use lightweight libraries like Zustand or Jotai for less complicated nation management requirements.
Understanding State Management in Next.js
State management refers to how your software handles and synchronizes records across special additives. In Next.Js 15, dealing with state efficiently ensures better overall performance, decreased re-renders, and progressed scalability.
Why is State Management Important?
Consistency: Ensure constant UI updates across components.
Performance: Reduce needless re-renders.
Scalability: Handle big-scale packages with complex records flows.
Developer Experience: Simplify debugging and maintainability.
Types of State in Next.js
Local State: Managed inside man or woman components.
Global State: Shared across additives and pages.
Server-Side State: Managed using server-rendering strategies.
URL State: Managed through query parameters.
Pro Tip: For large-scale applications, consider separating local and global state to prevent excessive re-renders.
Challenges in State Management
Overuse of global nation.
Difficulty debugging complicated dependencies.
State synchronization across consumer and server.
Maintaining overall performance with frequent kingdom updates.
Inefficient facts-fetching techniques.
When to Use Which State Management Approach?
Local State: For UI-particular states like toggles or modals.
Global State: For information shared across a couple of additives.
Server-Side State: For records fetched on the server and rendered statically.
Context API: For light-weight state management without outside libraries.
Insight: A hybrid technique frequently works high-quality to combine local, global, and server-aspect kingdoms strategically.
Core State Management Techniques in Next.js
Next.js supports multiple approaches to state management, depending on your application's size and complexity.
1. Local Component State
Local state is managed within individual components using React's built-in useState or useReducer hooks.
Ideal for managing small, isolated states.
Limited to a single component.
Simple and lightweight.
Key Points:
Keep it minimal.
Avoid prop drilling.
Use for UI-related states
Example: import { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( ); }
Quick Tip: Avoid using local state for data shared across multiple components.
2. Global State ManagementGlobal state handles data shared across multiple components or pages. Popular libraries include Redux, Zustand, and Jotai.
Centralized data store.
Suitable for large applications.
Enables better state synchronization.
Key Points:
Keep the state predictable.
Use middleware for side effects.
Avoid unnecessary global state
import create from 'zustand'; const useStore = create((set) => ({ count: 0, increase: () => set((state) => ({ count: state.count + 1 })) })); function Counter() { const { count, increase } = useStore(); return ; }Pro Tip: Choose lightweight libraries for simple use cases to reduce bundle size.
3. Server-Side State Management
Server-facet state is managed through functions like getServerSideProps and getStaticProps in Next.Js.
Fetch and manipulate facts on the server.
Pre-render pages with sparkling information.
Improves SEO and performance.
Key Points:
Use SSR handiest when necessary.
Avoid over-fetching statistics.
Keep API responses minimum.
Example:
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return { props: { data } };
}
Use server-side fetching only when absolutely necessary.
4. Context API for State Sharing
React's Context API permits international kingdom sharing without requiring
Suitable for small to medium-sized tasks.
Avoids prop drilling.
Works properly with useReducer for extra manipulation.
Key Points:
Keep contexts unique.
Avoid deep nesting.
Combine with useReducer for nation logic.
Pro Tip: Avoid the usage of Context for regularly updated states to save you overall performance bottlenecks.
Common issue in State Management
While coping with nation in Next.Js, developers often come upon not unusual issue:
Overusing Global State: Not each piece of data wishes to be worldwide.
Ignoring Performance Impacts: Excessive state updates can gradual down rendering.
Mixing Server-Side and Client-Side State Poorly: Leads to facts inconsistencies.
Skipping State Cleanup: Always easy up state whilst additives unmount.
Lack of Documentation: Poor documentation leads to confusion.
Key Suggestions:
Plan your state architecture earlier than implementation.
Use light-weight libraries whilst feasible.
Regularly audit nation dependencies.
Ensure clear documentation on your kingdom management approach.
State control is an ongoing undertaking to optimize and revisit your structure often.